https://cp-algorithms.com/data_structures/sqrt_decomposition.html
https://www.geeksforgeeks.org/sqrt-square-root-decomposition-technique-set-1-introduction/
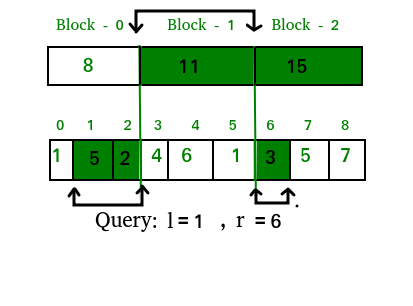
We can deal these type of queries by summing the data from the completely overlapped decomposed blocks lying in the query range and then summing elements one by one from the original array whose corresponding block is not completely overlapped by the query range.
So answer for above query in the described image will be : ans = 5 + 2 + 11 + 3 = 21
Time Complexity: Let’s consider a query [l = 1 and r = n-2] (n is the size of the array and has a 0 based indexing). Therefore, for this query exactly ( sqrt(n) – 2 ) blocks will be completely overlapped where as the first and last block will be partially overlapped with just one element left outside the overlapping range. So, the completely overlapped blocks can be summed up in ( sqrt(n) – 2 ) ~ sqrt(n) iterations, whereas first and last block are needed to be traversed one by one separately. But as we know that the number of elements in each block is at max sqrt(n), to sum up last block individually we need to make,
(sqrt(n)-1) ~ sqrt(n) iterations and same for the last block.
So, the overall Complexity = O(sqrt(n)) + O(sqrt(n)) + O(sqrt(n)) = O(3*sqrt(N)) = O(sqrt(n))
https://www.geeksforgeeks.org/sqrt-square-root-decomposition-technique-set-1-introduction/
Sqrt (or Square Root) Decomposition Technique is one of the most common query optimization technique used by competitive programmers. This technique helps us to reduce Time Complexity by a factor of sqrt(n).
The key concept of this technique is to decompose given array into small chunks specifically of size sqrt(n).
Let’s say we have an array of n elements and we decompose this array into small chunks of size sqrt(n). We will be having exactly sqrt(n) such chunks provided that n is a perfect square. Therefore, now our array on n elements is decomposed into sqrt(n) blocks, where each block contains sqrt(n) elements (assuming size of array is perfect square).
Let’s consider these chunks or blocks as an individual array each of which contains sqrt(n) elements and you have computed your desired answer(according to your problem) individually for all the chunks. Now, you need to answer certain queries asking you the answer for the elements in range l to r(l and r are starting and ending indices of the array) in the original n sized array.
Sqrt Decomposition Trick : As we have already precomputed the answer for all individual chunks and now we need to answer the queries in range l to r. Now we can simply combine the answers of the chunks that lie in between the range l to r in the original array. So, if we see carefully here we are jumping sqrt(n) steps at a time instead of jumping 1 step at a time as done in naive approach. Let’s just analyze its Time Complexity and implementation considering the below problem :-
Problem : Given an array of n elements. We need to answer q queries telling the sum of elements in range l to r in the array. Also the array is not static i.e the values are changed via some point update query. Range Sum Queries are of form : Q l r , where l is the starting index r is the ending index Point update Query is of form : U idx val, where idx is the index to update val is the updated value
Let us consider that we have an array of 9 elements.
A[] = {1, 5, 2, 4, 6, 1, 3, 5, 7}
A[] = {1, 5, 2, 4, 6, 1, 3, 5, 7}
Let’s decompose this array into sqrt(9) blocks, where each block will contain the sum of elements lying in it. Therefore now our decomposed array would look like this :
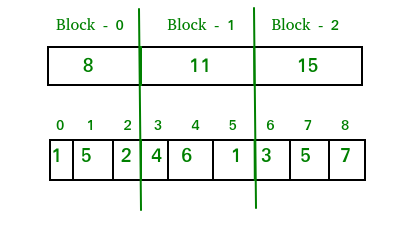
Till now we have constructed the decomposed array of sqrt(9) blocks and now we need to print the sum of elements in a given range. So first let’s see two basic types of overlap that a range query can have on our array :-
Range Query of type 1 (Given Range is on Block Boundaries) :
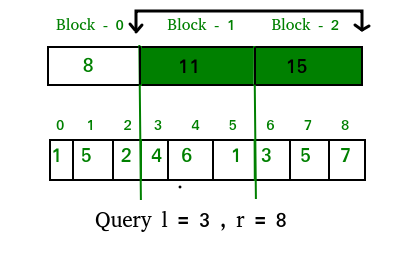
In this type the query, the range may totally cover the continuous sqrt blocks. So we can easily answer the sum of values in this range as the sum of completely overlapped blocks.
So answer for above query in the described image will be : ans = 11 + 15 = 26
Time Complexity: In the worst case our range can be 0 to n-1(where n is the size of array and assuming n to be a perfect square). In this case all the blocks are completely overlapped by our query range. Therefore,to answer this query we need to iterate over all the decomposed blocks for the array and we know that the number of blocks = sqrt(n). Hence, the complexity for this type of query will be O(sqrt(n)) in worst case.
So answer for above query in the described image will be : ans = 11 + 15 = 26
Time Complexity: In the worst case our range can be 0 to n-1(where n is the size of array and assuming n to be a perfect square). In this case all the blocks are completely overlapped by our query range. Therefore,to answer this query we need to iterate over all the decomposed blocks for the array and we know that the number of blocks = sqrt(n). Hence, the complexity for this type of query will be O(sqrt(n)) in worst case.
Range Query of type 2 (Given Range is NOT on boundaries)
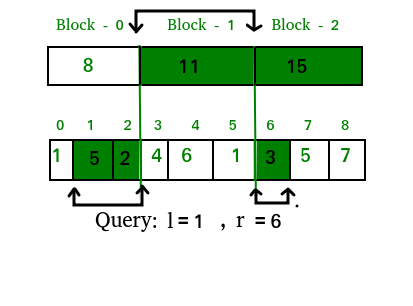
We can deal these type of queries by summing the data from the completely overlapped decomposed blocks lying in the query range and then summing elements one by one from the original array whose corresponding block is not completely overlapped by the query range.
So answer for above query in the described image will be : ans = 5 + 2 + 11 + 3 = 21
Time Complexity: Let’s consider a query [l = 1 and r = n-2] (n is the size of the array and has a 0 based indexing). Therefore, for this query exactly ( sqrt(n) – 2 ) blocks will be completely overlapped where as the first and last block will be partially overlapped with just one element left outside the overlapping range. So, the completely overlapped blocks can be summed up in ( sqrt(n) – 2 ) ~ sqrt(n) iterations, whereas first and last block are needed to be traversed one by one separately. But as we know that the number of elements in each block is at max sqrt(n), to sum up last block individually we need to make,
(sqrt(n)-1) ~ sqrt(n) iterations and same for the last block.
So, the overall Complexity = O(sqrt(n)) + O(sqrt(n)) + O(sqrt(n)) = O(3*sqrt(N)) = O(sqrt(n))