https://leetcode.com/problems/binary-search-tree-iterator/
https://leetcode.com/problems/binary-search-tree-iterator/discuss/52584/My-java-accepted-solution
X.
With parent instead of stack O(1) space
1. ⾃自⾏行行定义何为iterator
http://www.1point3acres.com/bbs/thread-142642-1-1.html
2. follow up 是不不让⽤用stack,node会加⼀一个parent;
3. preorder postorder
https://github.com/mintycc/OnlineJudge-Solutions/blob/master/untag/Binary%20Search%20Tree%20Iterator/BinarySearchTreeIterator.java
Implement an iterator over a binary search tree (BST). Your iterator will be initialized with the root node of a BST.
Calling
next()
will return the next smallest number in the BST.
Example:
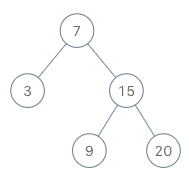
BSTIterator iterator = new BSTIterator(root); iterator.next(); // return 3 iterator.next(); // return 7 iterator.hasNext(); // return true iterator.next(); // return 9 iterator.hasNext(); // return true iterator.next(); // return 15 iterator.hasNext(); // return true iterator.next(); // return 20 iterator.hasNext(); // return false
Note:
next()
andhasNext()
should run in average O(1) time and uses O(h) memory, where h is the height of the tree.- You may assume that
next()
call will always be valid, that is, there will be at least a next smallest number in the BST whennext()
is called
I use Stack to store directed left children from root.
When next() be called, I just pop one element and process its right child as new root.
The code is pretty straightforward.
When next() be called, I just pop one element and process its right child as new root.
The code is pretty straightforward.
So this can satisfy O(h) memory, hasNext() in O(1) time,
But next() is O(h) time.
But next() is O(h) time.
private Stack<TreeNode> stack = new Stack<TreeNode>();
public BSTIterator(TreeNode root) {
pushAll(root);
}
/** @return whether we have a next smallest number */
public boolean hasNext() {
return !stack.isEmpty();
}
/** @return the next smallest number */
public int next() {
TreeNode tmpNode = stack.pop();
pushAll(tmpNode.right);
return tmpNode.val;
}
private void pushAll(TreeNode node) {
for (; node != null; stack.push(node), node = node.left);
}
https://leetcode.com/problems/binary-search-tree-iterator/discuss/52584/My-java-accepted-solution
Stack<TreeNode> stack = null ;
TreeNode current = null ;
public BSTIterator(TreeNode root) {
current = root;
stack = new Stack<> ();
}
/** @return whether we have a next smallest number */
public boolean hasNext() {
return !stack.isEmpty() || current != null;
}
/** @return the next smallest number */
public int next() {
while (current != null) {
stack.push(current);
current = current.left ;
}
TreeNode t = stack.pop() ;
current = t.right ;
return t.val ;
}
X.
With parent instead of stack O(1) space
1. ⾃自⾏行行定义何为iterator
http://www.1point3acres.com/bbs/thread-142642-1-1.html
2. follow up 是不不让⽤用stack,node会加⼀一个parent;
3. preorder postorder
https://github.com/mintycc/OnlineJudge-Solutions/blob/master/untag/Binary%20Search%20Tree%20Iterator/BinarySearchTreeIterator.java
static class BinarySearchTree {
Node root;
// Iterator part
class BSTIterator implements Iterator<Node> {
Node last = null;
BSTIterator(Node root) {
if (root == null) return;
last = root;
while (last.left != null)
last = last.left;
}
public boolean hasNext() {
return last != null;
}
public Node next() {
Node cur = last;
last = findSuccessor(last);
return cur;
}
private Node findSuccessor(Node root) {
if (root == null) return null;
if (root.right != null) {
Node tmp = root.right;
while (tmp.left != null) tmp = tmp.left;
return tmp;
}
Node father = root.parent;
Node child = root;
while (father != null && father.left != child) {
child = father;
father = father.parent;
}
return father;
}
}
Iterator<Node> iterator() {
BSTIterator iter = new BSTIterator(root);
return iter;
}
// Given a binary search tree and a number, inserts a new node
// with the given number in the correct place in the tree
void insert(int key) {
// 1. If the tree is empty, create the root
if(this.root == null) {
this.root = new Node(key);
return;
}
// 2) Otherwise, create a node with the key
// and traverse down the tree to find where to
// to insert the new node
Node currentNode = this.root;
Node newNode = new Node(key);
while(currentNode != null) {
if(key < currentNode.key) {
if(currentNode.left == null) {
currentNode.left = newNode;
newNode.parent = currentNode;
break;
} else {
currentNode = currentNode.left;
}
} else {
if(currentNode.right == null) {
currentNode.right = newNode;
newNode.parent = currentNode;
break;
} else {
currentNode = currentNode.right;
}
}
}
}
// Return a reference to a node in the BST by its key.
// Use this method when you need a node to test your
// findInOrderSuccessor method on
Node getNodeByKey(int key) {
Node currentNode = this.root;
while(currentNode != null) {
if(key == currentNode.key) {
return currentNode;
}
if(key < currentNode.key) {
currentNode = currentNode.left;
} else {
currentNode = currentNode.right;
}
}
return null;
}
}