https://leetcode.com/problems/populating-next-right-pointers-in-each-node/
X. Level Order traverse
https://leetcode.com/problems/populating-next-right-pointers-in-each-node/discuss/37484/7-lines-iterative-real-O(1)-space
https://leetcode.com/problems/populating-next-right-pointers-in-each-node/discuss/37473/My-recursive-solution(Java)
I wonder if the root.right is null, the code
- no need
You are given a perfect binary tree where all leaves are on the same level, and every parent has two children. The binary tree has the following definition:
struct Node { int val; Node *left; Node *right; Node *next; }
Populate each next pointer to point to its next right node. If there is no next right node, the next pointer should be set to
NULL
.
Initially, all next pointers are set to
NULL
.
Example:
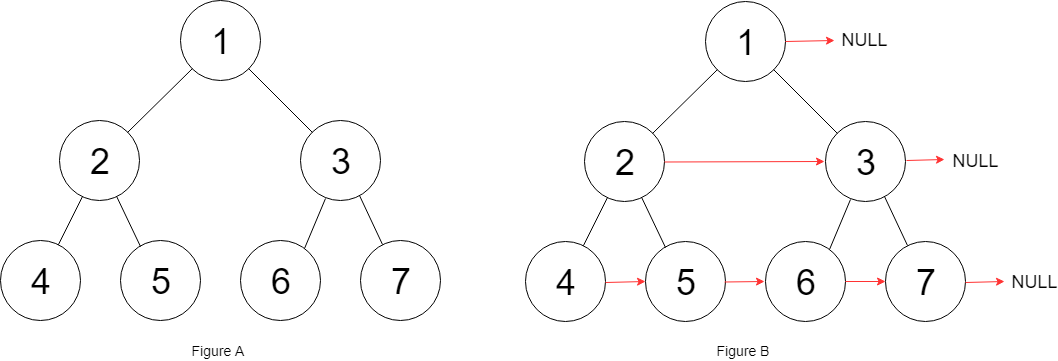
Note:
- You may only use constant extra space.
- Recursive approach is fine, implicit stack space does not count as extra space for this problem.
X. Level Order traverse
public void connect(TreeLinkNode root) {
TreeLinkNode level_start=root;
while(level_start!=null){
TreeLinkNode cur=level_start;
while(cur!=null){
if(cur.left!=null) cur.left.next=cur.right;
if(cur.right!=null && cur.next!=null) cur.right.next=cur.next.left;//\\key
cur=cur.next;
}
level_start=level_start.left;
}
}
public void connect(TreeLinkNode root) {
if(root==null) return;
TreeLinkNode cur = root;
TreeLinkNode nextLeftmost = null;
while(cur.left!=null){
nextLeftmost = cur.left; // save the start of next level
while(cur!=null){
cur.left.next=cur.right;
cur.right.next = cur.next==null? null : cur.next.left;
cur=cur.next;
}
cur=nextLeftmost; // point to next level
}
}
Simply do it level by level, using the next
-pointers of the current level to go through the current level and set the next
-pointers of the next level.
I say "real" O(1) space because of the many recursive solutions ignoring that recursion management needs space.
def connect(self, root):
while root and root.left:
next = root.left
while root:
root.left.next = root.right
root.right.next = root.next and root.next.left
root = root.next
root = next
Solution1 : O(1) space complexity
public Node connect(Node root) {
if(root == null) return root;
Node dummy = new Node(0);
dummy.next = root;
Node pre = root;
while(root.left != null){
Node preRight = null;
while(root != null){
if(preRight != null) preRight.next = root.left;
root.left.next = root.right;
preRight = root.right;
root = root.next;
}
root = pre.left;
pre = root;
}
return dummy.next;
}
X. Recursion, pre-order traversehttps://leetcode.com/problems/populating-next-right-pointers-in-each-node/discuss/37473/My-recursive-solution(Java)
I wonder if the root.right is null, the code
root.right.next = root.next.left;
will cause a error? Why don't need to ensure root.right != null
first?public void connect(TreeLinkNode root) {
if(root == null)
return;
if(root.left != null){
root.left.next = root.right;
if(root.next != null)
root.right.next = root.next.left;
}
connect(root.left);
connect(root.right);
}
X. https://leetcode.com/problems/populating-next-right-pointers-in-each-node/discuss/257083/Simple-Java-beats-100-recursive-easy-understanding-with-explanation- no need
For each node, we will connect all the links between the left subtree and the right. For example, for the following tree and the node 1, we will connect the following links:
1
2 ----> 3
4 5 ->6 7
8 8 8 8->8 8 8 8
And do it recursively for each node.
public Node connect(Node root) {
helper(root);
return root;
}
public void helper(Node node){
if(node == null) return;
Node left = node.left;
Node right = node.right;
while(left != null){
left.next = right;
left = left.right;
right = right.left;
}
helper(node.left);
helper(node.right);
return;
}
X. https://leetcode.com/problems/populating-next-right-pointers-in-each-node/discuss/37472/A-simple-accepted-solution
Solution2 : O(n) space complexity
public Node connect(Node root) {
if(root == null) return root;
List<Node> list = new ArrayList<>();
list.add(root);
while(!list.isEmpty()){
int size = list.size();
Node pre = null;
for(int i = 0;i<size;i++){
Node curr = list.remove(0);
if(pre == null){
pre = curr;
}else{
pre.next = curr;
pre = curr;
}
if(curr.left != null) list.add(curr.left);
if(curr.right != null) list.add(curr.right);
}
}
return root;
}