Trie Serialization
http://shibaili.blogspot.com/2018/11/428-serialize-and-deserialize-n-ary-tree.html
X. Level Order traverse
http://shibaili.blogspot.com/2018/11/428-serialize-and-deserialize-n-ary-tree.html
X. Pre-order
https://github.com/Cee/Leetcode/blob/master/428%20-%20Serialize%20and%20Deserialize%20N-ary%20Tree.java
https://segmentfault.com/a/1190000016803936
Serialize and Deserialize an N-ary Tree - GeeksforGeeks
Given an N-ary tree where every node has at-most N children. How to serialize and deserialze it? Serialization is to store tree in a file so that it can be later restored. The structure of tree must be maintained. Deserialization is reading tree back from file.
In an N-ary tree, there are no designated left and right children. An N-ary tree is represented by storing an array or list of child pointers with every node.
The idea is to store an ‘end of children’ marker with every node. The following diagram shows serialization where ‘)’ is used as end of children marker. The diagram is taken from here.
#define MARKER ')'
I guess you don't care about "which" child it is...You have an arbitrary number of children and that's it. If you care about index of the child then yes you cannot know.
https://gist.github.com/ajaynitt/ce01da0d3cfdd5c8db1c
https://mameko.gitbooks.io/pan-s-algorithm-notes/content/26-tree/serilization-and-deserialize-n-ary-tree.html
http://ideone.com/TO4dEs
http://eli.thegreenplace.net/2011/09/29/an-interesting-tree-serialization-algorithm-from-dwarf
http://interviewpractise.blogspot.com/2014/11/serialize-and-deserialize-n-ary-tree.html
Related: http://massivealgorithms.blogspot.com/2014/09/serializationdeserialization-of-binary.html
Read full article from Serialize and Deserialize an N-ary Tree - GeeksforGeeks
http://shibaili.blogspot.com/2018/11/428-serialize-and-deserialize-n-ary-tree.html
Serialization is the process of converting a data structure or object into a sequence of bits so that it can be stored in a file or memory buffer, or transmitted across a network connection link to be reconstructed later in the same or another computer environment.
Design an algorithm to serialize and deserialize an N-ary tree. An N-ary tree is a rooted tree in which each node has no more than N children. There is no restriction on how your serialization/deserialization algorithm should work. You just need to ensure that an N-ary tree can be serialized to a string and this string can be deserialized to the original tree structure.
For example, you may serialize the following
3-ary
tree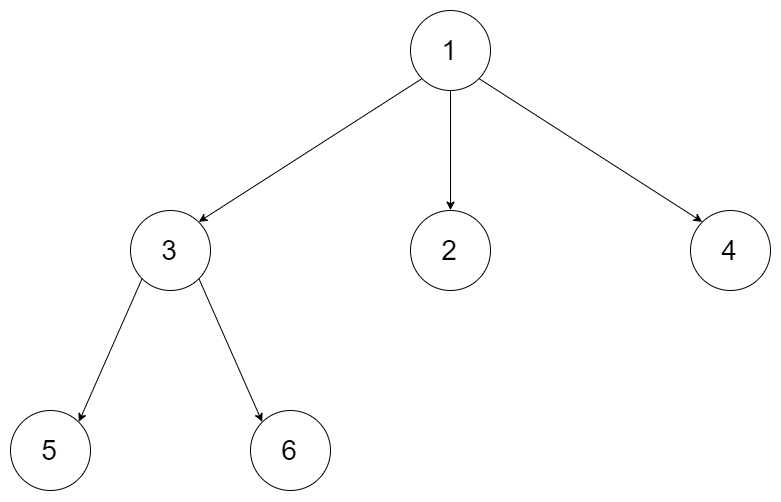
as
[1 [3[5 6] 2 4]]
. You do not necessarily need to follow this format, so please be creative and come up with different approaches yourself.
Note:
N
is in the range of[1, 1000]
- Do not use class member/global/static variables to store states. Your serialize and deserialize algorithms should be stateless.
X. Level Order traverse
http://shibaili.blogspot.com/2018/11/428-serialize-and-deserialize-n-ary-tree.html
public
String serialize(Node root) {
if
(root ==
null
)
return
""
;
Queue<Node> que =
new
LinkedList<>();
StringBuilder sb =
new
StringBuilder();
sb.append(Integer.toString(root.val)).append(
",#,"
);
que.add(root);
while
(!que.isEmpty()) {
Node node = que.poll();
for
(Node n : node.children) {
sb.append(Integer.toString(n.val)).append(
","
);
que.add(n);
}
sb.append(
"#,"
);
}
return
sb.toString();
}
// Decodes your encoded data to tree.
public
Node deserialize(String data) {
if
(data.length() ==
0
)
return
null
;
String[] s = data.split(
","
);
Queue<Node> que =
new
LinkedList<>();
Node root =
new
Node(Integer.parseInt(s[
0
]),
new
ArrayList<Node>());
que.add(root);
int
i =
1
;
while
(!que.isEmpty()) {
Node node = que.poll();
i++;
while
(!s[i].equals(
"#"
)) {
Node c =
new
Node(Integer.parseInt(s[i]),
new
ArrayList<>());
node.children.add(c);
que.add(c);
i++;
}
}
return
root;
}
X. Pre-order
https://github.com/Cee/Leetcode/blob/master/428%20-%20Serialize%20and%20Deserialize%20N-ary%20Tree.java
public String serialize(Node root) {
if (root == null) { return ""; }
StringBuilder sb = new StringBuilder();
sb.append(root.val);
sb.append(",");
sb.append(root.children.size());
sb.append("#");
for (Node c: root.children) {
sb.append(serialize(c));
}
return sb.toString();
}
class Rep {
Node n;
int end;
}
// Decodes your encoded data to tree.
public Node deserialize(String data) {
if (data.length() == 0) { return null; }
return parse(data, 0, data.length() - 1).n;
}
private Rep parse(String data, int start, int end) {
if (start >= end) { return null; }
int comma = data.indexOf(",", start);
int split = data.indexOf("#", start);
int val = Integer.valueOf(data.substring(start, comma));
int childNum = Integer.valueOf(data.substring(comma + 1, split));
Node n = new Node();
n.val = val;
List<Node> children = new ArrayList<>();
int last = split;
for (int i = 0; i < childNum; i++) {
Rep rep = parse(data, last + 1, end);
last = rep.end;
children.add(rep.n);
}
n.children = children;
Rep r = new Rep();
r.n = n;
r.end = last;
return r;
}
Serialize and Deserialize an N-ary Tree - GeeksforGeeks
Given an N-ary tree where every node has at-most N children. How to serialize and deserialze it? Serialization is to store tree in a file so that it can be later restored. The structure of tree must be maintained. Deserialization is reading tree back from file.
In an N-ary tree, there are no designated left and right children. An N-ary tree is represented by storing an array or list of child pointers with every node.
The idea is to store an ‘end of children’ marker with every node. The following diagram shows serialization where ‘)’ is used as end of children marker. The diagram is taken from here.
#define MARKER ')'
void
serialize(Node *root,
FILE
*fp)
{
// Base case
if
(root == NULL)
return
;
// Else, store current node and recur for its children
fprintf
(fp,
"%c "
, root->key);
for
(
int
i = 0; i < N && root->child[i]; i++)
serialize(root->child[i], fp);
// Store marker at the end of children
fprintf
(fp,
"%c "
, MARKER);
}
// This function constructs N-ary tree from a file pointed by 'fp'.
// This functionr returns 0 to indicate that the next item is a valid
// tree key. Else returns 0
int
deSerialize(Node *&root,
FILE
*fp)
{
// Read next item from file. If theere are no more items or next
// item is marker, then return 1 to indicate same
char
val;
if
( !
fscanf
(fp,
"%c "
, &val) || val == MARKER )
return
1;
// Else create node with this item and recur for children
root = newNode(val);
for
(
int
i = 0; i < N; i++)
if
(deSerialize(root->child[i], fp))
break
;
// Finally return 0 for successful finish
return
0;
}
https://gist.github.com/ajaynitt/ce01da0d3cfdd5c8db1c
https://mameko.gitbooks.io/pan-s-algorithm-notes/content/26-tree/serilization-and-deserialize-n-ary-tree.html
public String serialize(NAryNode root) {
if (root == null) {
return "";
}
StringBuilder sb = new StringBuilder();
dfsHelper(sb, root);
return sb.toString();
}
private void dfsHelper(StringBuilder sb, NAryNode root) {
if (root == null) {
return;
}
// sb.append("(");// 可以省一些空间
sb.append(root.label);
for (NAryNode child : root.children) {
dfsHelper(sb, child);
}
sb.append(")");
}
int index = 0;
public NAryNode deserialize(String input) {
if (input == null || input.isEmpty() || index >= input.length()) {
return null;
}
// if (input.charAt(index) == '(') {//省了空间以后就不用跳过'('了
// index++;// skip '('
NAryNode node = new NAryNode(input.charAt(index));// create node
index++;// go to next char
while (input.charAt(index) != ')') { // if not == ')', has child
node.children.add(deserialize(input));// so create child node recursively
}
index++;// no child / finished creating child go to next char
return node;
// }
// return null;
}
public static void main(String[] args) {
SerializeDeserializeNaryTree sol = new SerializeDeserializeNaryTree();
NAryNode a = new NAryNode('a');
NAryNode b = new NAryNode('b');
NAryNode c = new NAryNode('c');
NAryNode d = new NAryNode('d');
NAryNode e = new NAryNode('e');
NAryNode f = new NAryNode('f');
NAryNode g = new NAryNode('g');
NAryNode h = new NAryNode('h');
NAryNode i = new NAryNode('i');
NAryNode j = new NAryNode('j');
NAryNode k = new NAryNode('k');
a.children.add(b);
a.children.add(c);
a.children.add(d);
b.children.add(e);
b.children.add(f);
f.children.add(k);
d.children.add(g);
d.children.add(h);
d.children.add(i);
d.children.add(j);
String input = sol.serialize(a);
System.out.println(input);
NAryNode res = sol.deserialize(input);
// 省空间的输出:abe)fk)))c)dg)h)i)j)))
// 不省的输出:(a(b(e)(f(k)))(c)(d(g)(h)(i)(j)))
}
}
class NAryNode {
char label;
List<NAryNode> children;}
http://ideone.com/TO4dEs
http://eli.thegreenplace.net/2011/09/29/an-interesting-tree-serialization-algorithm-from-dwarf
http://interviewpractise.blogspot.com/2014/11/serialize-and-deserialize-n-ary-tree.html
Related: http://massivealgorithms.blogspot.com/2014/09/serializationdeserialization-of-binary.html
Read full article from Serialize and Deserialize an N-ary Tree - GeeksforGeeks